Function to check if object-dtype column value is float or string
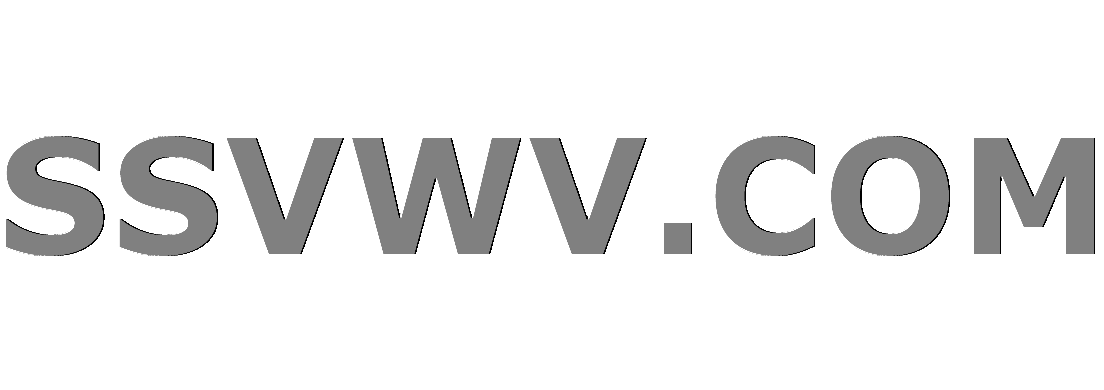
Multi tool use
I am trying to write a function which is equal to isnumber[column] function in excel
dataset:
feature1 feature2 feature3
123 1.07 1
231 2.08 3
122 ab 4
111 3.04 6
555 cde 8
feature1: integer dtype
feature2: object dtype
feature3: integer dtype
I tried this piece of code
for item in df.feature2.iteritems():
if isinstance(item, float):
print('yes')
else:
print('no')
I got the result as
no
no
no
no
no
But i want the result as
yes
yes
no
yes
no
When i tried to check the type of individual feature2 values, this is what see
type(df.feature2[0]) = str
type(df.feature2[1]) = str
type(df.feature2[2]) = str
type(df.feature2[3]) = str
type(df.feature2[4]) = str
But clearly 0,1,3 should be shown as float, but they show up as str
What am i doing wrong?
python python-3.x python-2.7 user-defined-types isinstance
add a comment |
I am trying to write a function which is equal to isnumber[column] function in excel
dataset:
feature1 feature2 feature3
123 1.07 1
231 2.08 3
122 ab 4
111 3.04 6
555 cde 8
feature1: integer dtype
feature2: object dtype
feature3: integer dtype
I tried this piece of code
for item in df.feature2.iteritems():
if isinstance(item, float):
print('yes')
else:
print('no')
I got the result as
no
no
no
no
no
But i want the result as
yes
yes
no
yes
no
When i tried to check the type of individual feature2 values, this is what see
type(df.feature2[0]) = str
type(df.feature2[1]) = str
type(df.feature2[2]) = str
type(df.feature2[3]) = str
type(df.feature2[4]) = str
But clearly 0,1,3 should be shown as float, but they show up as str
What am i doing wrong?
python python-3.x python-2.7 user-defined-types isinstance
add a comment |
I am trying to write a function which is equal to isnumber[column] function in excel
dataset:
feature1 feature2 feature3
123 1.07 1
231 2.08 3
122 ab 4
111 3.04 6
555 cde 8
feature1: integer dtype
feature2: object dtype
feature3: integer dtype
I tried this piece of code
for item in df.feature2.iteritems():
if isinstance(item, float):
print('yes')
else:
print('no')
I got the result as
no
no
no
no
no
But i want the result as
yes
yes
no
yes
no
When i tried to check the type of individual feature2 values, this is what see
type(df.feature2[0]) = str
type(df.feature2[1]) = str
type(df.feature2[2]) = str
type(df.feature2[3]) = str
type(df.feature2[4]) = str
But clearly 0,1,3 should be shown as float, but they show up as str
What am i doing wrong?
python python-3.x python-2.7 user-defined-types isinstance
I am trying to write a function which is equal to isnumber[column] function in excel
dataset:
feature1 feature2 feature3
123 1.07 1
231 2.08 3
122 ab 4
111 3.04 6
555 cde 8
feature1: integer dtype
feature2: object dtype
feature3: integer dtype
I tried this piece of code
for item in df.feature2.iteritems():
if isinstance(item, float):
print('yes')
else:
print('no')
I got the result as
no
no
no
no
no
But i want the result as
yes
yes
no
yes
no
When i tried to check the type of individual feature2 values, this is what see
type(df.feature2[0]) = str
type(df.feature2[1]) = str
type(df.feature2[2]) = str
type(df.feature2[3]) = str
type(df.feature2[4]) = str
But clearly 0,1,3 should be shown as float, but they show up as str
What am i doing wrong?
python python-3.x python-2.7 user-defined-types isinstance
python python-3.x python-2.7 user-defined-types isinstance
edited Nov 21 '18 at 18:52
Sai Sumanth
asked Nov 21 '18 at 18:26


Sai SumanthSai Sumanth
313
313
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
Iteritems is returning a tuple, ((123, '1.07'), 1.07)
and since you want to loop over each value try the below code.
You just need to remove .iteritems()
and it will work like a charm.
df['feature2']=[1.07,2.08,'ab',3.04,'cde']
for item in df.feature2:
if isinstance(item,float):
print('yes')
else:
print('no')
Here is your output:
yes
yes
no
yes
no
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
add a comment |
Try this:
for i in range(len(df["feature2"])):
test = df.loc[i,"feature2"]
if isinstance(test, float):
print('yes')
else:
print('no')
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
add a comment |
This is because iteritems()
returns a tuple which is the (index, value)
.
So you are trying to check for example if (0, 1.07)
or (1, 2.08)
are of type float, which they aren't of course.
It should work if you change df.feature2.iteritems()
to df.feature2.values
:)
add a comment |
You can do something like this:
from pandas import DataFrame as df
columns = ['feature1', 'feature2', 'feature3']
data = [[123, 1.07, 1],
[231, 2.08, 3],
[122, 'ab', 4],
[111, 3.04, 6],
[555, 'cde', 8]]
df_ = df(data, columns=columns)
types =
for k in df_:
a = set(type(m) for m in df_[k])
if len(a) > 1:
types.append({k: 'object'})
else:
types.append({k: str(list(a)[0].__name__)})
print(types)
Output:
[{'feature1': 'int'}, {'feature2': 'object'}, {'feature3': 'int'}]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418392%2ffunction-to-check-if-object-dtype-column-value-is-float-or-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Iteritems is returning a tuple, ((123, '1.07'), 1.07)
and since you want to loop over each value try the below code.
You just need to remove .iteritems()
and it will work like a charm.
df['feature2']=[1.07,2.08,'ab',3.04,'cde']
for item in df.feature2:
if isinstance(item,float):
print('yes')
else:
print('no')
Here is your output:
yes
yes
no
yes
no
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
add a comment |
Iteritems is returning a tuple, ((123, '1.07'), 1.07)
and since you want to loop over each value try the below code.
You just need to remove .iteritems()
and it will work like a charm.
df['feature2']=[1.07,2.08,'ab',3.04,'cde']
for item in df.feature2:
if isinstance(item,float):
print('yes')
else:
print('no')
Here is your output:
yes
yes
no
yes
no
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
add a comment |
Iteritems is returning a tuple, ((123, '1.07'), 1.07)
and since you want to loop over each value try the below code.
You just need to remove .iteritems()
and it will work like a charm.
df['feature2']=[1.07,2.08,'ab',3.04,'cde']
for item in df.feature2:
if isinstance(item,float):
print('yes')
else:
print('no')
Here is your output:
yes
yes
no
yes
no
Iteritems is returning a tuple, ((123, '1.07'), 1.07)
and since you want to loop over each value try the below code.
You just need to remove .iteritems()
and it will work like a charm.
df['feature2']=[1.07,2.08,'ab',3.04,'cde']
for item in df.feature2:
if isinstance(item,float):
print('yes')
else:
print('no')
Here is your output:
yes
yes
no
yes
no
edited Nov 21 '18 at 19:01
answered Nov 21 '18 at 18:42


Ankur GulatiAnkur Gulati
14510
14510
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
add a comment |
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
If it helps, please care to accept and upvote the answer. Thanks :)
– Ankur Gulati
Nov 21 '18 at 18:43
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
Sorry, it did not work. I'm not sure why
– Sai Sumanth
Nov 21 '18 at 18:58
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
@SaiSumanth Can you tell me what's the error? I included the data frame creation line that I used for testing and it is working from me. Also, I am using Python3
– Ankur Gulati
Nov 21 '18 at 19:03
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
Its working now, actually my features values were in 'float string' type instead of float type. Thanks
– Sai Sumanth
Nov 21 '18 at 19:20
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
No Problem. Please accept the answer.
– Ankur Gulati
Nov 21 '18 at 19:30
add a comment |
Try this:
for i in range(len(df["feature2"])):
test = df.loc[i,"feature2"]
if isinstance(test, float):
print('yes')
else:
print('no')
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
add a comment |
Try this:
for i in range(len(df["feature2"])):
test = df.loc[i,"feature2"]
if isinstance(test, float):
print('yes')
else:
print('no')
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
add a comment |
Try this:
for i in range(len(df["feature2"])):
test = df.loc[i,"feature2"]
if isinstance(test, float):
print('yes')
else:
print('no')
Try this:
for i in range(len(df["feature2"])):
test = df.loc[i,"feature2"]
if isinstance(test, float):
print('yes')
else:
print('no')
answered Nov 21 '18 at 18:37
Ellie HannaEllie Hanna
585
585
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
add a comment |
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
bear in mind that this just tests for floats - if you want any number, float or integer, you'd have to change the third line to if isinstance(test, float) or isinstance(test, int):
– Ellie Hanna
Nov 21 '18 at 18:39
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
okay i will try
– Sai Sumanth
Nov 21 '18 at 18:40
add a comment |
This is because iteritems()
returns a tuple which is the (index, value)
.
So you are trying to check for example if (0, 1.07)
or (1, 2.08)
are of type float, which they aren't of course.
It should work if you change df.feature2.iteritems()
to df.feature2.values
:)
add a comment |
This is because iteritems()
returns a tuple which is the (index, value)
.
So you are trying to check for example if (0, 1.07)
or (1, 2.08)
are of type float, which they aren't of course.
It should work if you change df.feature2.iteritems()
to df.feature2.values
:)
add a comment |
This is because iteritems()
returns a tuple which is the (index, value)
.
So you are trying to check for example if (0, 1.07)
or (1, 2.08)
are of type float, which they aren't of course.
It should work if you change df.feature2.iteritems()
to df.feature2.values
:)
This is because iteritems()
returns a tuple which is the (index, value)
.
So you are trying to check for example if (0, 1.07)
or (1, 2.08)
are of type float, which they aren't of course.
It should work if you change df.feature2.iteritems()
to df.feature2.values
:)
answered Nov 21 '18 at 18:38


hmajid2301hmajid2301
178
178
add a comment |
add a comment |
You can do something like this:
from pandas import DataFrame as df
columns = ['feature1', 'feature2', 'feature3']
data = [[123, 1.07, 1],
[231, 2.08, 3],
[122, 'ab', 4],
[111, 3.04, 6],
[555, 'cde', 8]]
df_ = df(data, columns=columns)
types =
for k in df_:
a = set(type(m) for m in df_[k])
if len(a) > 1:
types.append({k: 'object'})
else:
types.append({k: str(list(a)[0].__name__)})
print(types)
Output:
[{'feature1': 'int'}, {'feature2': 'object'}, {'feature3': 'int'}]
add a comment |
You can do something like this:
from pandas import DataFrame as df
columns = ['feature1', 'feature2', 'feature3']
data = [[123, 1.07, 1],
[231, 2.08, 3],
[122, 'ab', 4],
[111, 3.04, 6],
[555, 'cde', 8]]
df_ = df(data, columns=columns)
types =
for k in df_:
a = set(type(m) for m in df_[k])
if len(a) > 1:
types.append({k: 'object'})
else:
types.append({k: str(list(a)[0].__name__)})
print(types)
Output:
[{'feature1': 'int'}, {'feature2': 'object'}, {'feature3': 'int'}]
add a comment |
You can do something like this:
from pandas import DataFrame as df
columns = ['feature1', 'feature2', 'feature3']
data = [[123, 1.07, 1],
[231, 2.08, 3],
[122, 'ab', 4],
[111, 3.04, 6],
[555, 'cde', 8]]
df_ = df(data, columns=columns)
types =
for k in df_:
a = set(type(m) for m in df_[k])
if len(a) > 1:
types.append({k: 'object'})
else:
types.append({k: str(list(a)[0].__name__)})
print(types)
Output:
[{'feature1': 'int'}, {'feature2': 'object'}, {'feature3': 'int'}]
You can do something like this:
from pandas import DataFrame as df
columns = ['feature1', 'feature2', 'feature3']
data = [[123, 1.07, 1],
[231, 2.08, 3],
[122, 'ab', 4],
[111, 3.04, 6],
[555, 'cde', 8]]
df_ = df(data, columns=columns)
types =
for k in df_:
a = set(type(m) for m in df_[k])
if len(a) > 1:
types.append({k: 'object'})
else:
types.append({k: str(list(a)[0].__name__)})
print(types)
Output:
[{'feature1': 'int'}, {'feature2': 'object'}, {'feature3': 'int'}]
answered Nov 21 '18 at 19:13


Chiheb NexusChiheb Nexus
5,31631829
5,31631829
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418392%2ffunction-to-check-if-object-dtype-column-value-is-float-or-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
C9xjv6U9GQma5q,smdT,WTGDmQS8BROwAFa4 z,I3,oyq,JTTc 8p1uvP2Jy48M