Why can't while(flag){} end
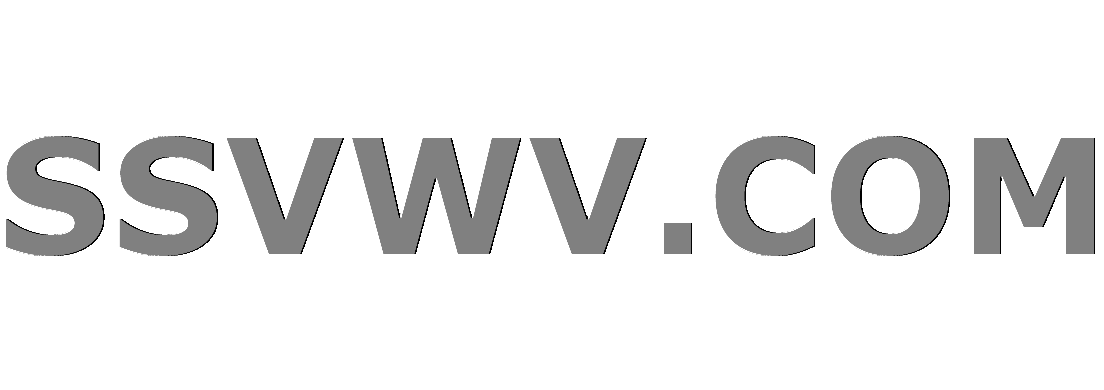
Multi tool use
code like this:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
Thread.sleep(100)
}
println("finish")
}
the run result is :
time over
finish
The program is over
if change to:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
//Do nothing
}
println("finish")
}
"finish" cannot be print,The program is stuck. Why is that?
java kotlin
add a comment |
code like this:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
Thread.sleep(100)
}
println("finish")
}
the run result is :
time over
finish
The program is over
if change to:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
//Do nothing
}
println("finish")
}
"finish" cannot be print,The program is stuck. Why is that?
java kotlin
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09
add a comment |
code like this:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
Thread.sleep(100)
}
println("finish")
}
the run result is :
time over
finish
The program is over
if change to:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
//Do nothing
}
println("finish")
}
"finish" cannot be print,The program is stuck. Why is that?
java kotlin
code like this:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
Thread.sleep(100)
}
println("finish")
}
the run result is :
time over
finish
The program is over
if change to:
fun main(args: Array<String>) {
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
while (flag) {
//Do nothing
}
println("finish")
}
"finish" cannot be print,The program is stuck. Why is that?
java kotlin
java kotlin
asked Nov 21 '18 at 5:42


Void YoungVoid Young
235
235
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09
add a comment |
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09
add a comment |
3 Answers
3
active
oldest
votes
Because you are getting a cached version of your flag. Take a look at the volatile keyword.
Basically here you are updating the flag in one thread, but the other is still seeing is own cached version of the flag.
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
add a comment |
The problem is first thread need time to set your flag is false but while loop execute before thread finish that means still your flag is true so that its never get false.
we need to set flow after thread finish then check while condition for that use thread sleep.
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
Thread.sleep(3000)
while (flag) {
}
println("finish")
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
add a comment |
When the variable flag is not declared volatile
, the compiler sometimes (depends on the one used) optimises your while loop code to the following :
if (condition) {
while (true) {
// Do nothing
}
}
Refer this link for more. Here is a similar SO question.
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405872%2fwhy-cant-whileflag-end%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Because you are getting a cached version of your flag. Take a look at the volatile keyword.
Basically here you are updating the flag in one thread, but the other is still seeing is own cached version of the flag.
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
add a comment |
Because you are getting a cached version of your flag. Take a look at the volatile keyword.
Basically here you are updating the flag in one thread, but the other is still seeing is own cached version of the flag.
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
add a comment |
Because you are getting a cached version of your flag. Take a look at the volatile keyword.
Basically here you are updating the flag in one thread, but the other is still seeing is own cached version of the flag.
Because you are getting a cached version of your flag. Take a look at the volatile keyword.
Basically here you are updating the flag in one thread, but the other is still seeing is own cached version of the flag.
answered Nov 21 '18 at 5:44


alerootaleroot
55.3k21143189
55.3k21143189
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
add a comment |
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
2
2
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Ah, you beat me by 2 seconds for the same answer :)
– PradyumanDixit
Nov 21 '18 at 5:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:45
add a comment |
The problem is first thread need time to set your flag is false but while loop execute before thread finish that means still your flag is true so that its never get false.
we need to set flow after thread finish then check while condition for that use thread sleep.
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
Thread.sleep(3000)
while (flag) {
}
println("finish")
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
add a comment |
The problem is first thread need time to set your flag is false but while loop execute before thread finish that means still your flag is true so that its never get false.
we need to set flow after thread finish then check while condition for that use thread sleep.
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
Thread.sleep(3000)
while (flag) {
}
println("finish")
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
add a comment |
The problem is first thread need time to set your flag is false but while loop execute before thread finish that means still your flag is true so that its never get false.
we need to set flow after thread finish then check while condition for that use thread sleep.
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
Thread.sleep(3000)
while (flag) {
}
println("finish")
The problem is first thread need time to set your flag is false but while loop execute before thread finish that means still your flag is true so that its never get false.
we need to set flow after thread finish then check while condition for that use thread sleep.
var flag = true
Thread {
Thread.sleep(2000)
println("time over")
flag = false
}.start()
Thread.sleep(3000)
while (flag) {
}
println("finish")
answered Nov 21 '18 at 5:49


sasikumarsasikumar
7,70011226
7,70011226
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
add a comment |
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
So why the "time over" is also not printing in 2nd case of OP?
– Amit Bera
Nov 21 '18 at 5:57
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
println("time over") is printing but not printing "finish" in 2nd case
– sasikumar
Nov 21 '18 at 6:02
add a comment |
When the variable flag is not declared volatile
, the compiler sometimes (depends on the one used) optimises your while loop code to the following :
if (condition) {
while (true) {
// Do nothing
}
}
Refer this link for more. Here is a similar SO question.
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
add a comment |
When the variable flag is not declared volatile
, the compiler sometimes (depends on the one used) optimises your while loop code to the following :
if (condition) {
while (true) {
// Do nothing
}
}
Refer this link for more. Here is a similar SO question.
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
add a comment |
When the variable flag is not declared volatile
, the compiler sometimes (depends on the one used) optimises your while loop code to the following :
if (condition) {
while (true) {
// Do nothing
}
}
Refer this link for more. Here is a similar SO question.
When the variable flag is not declared volatile
, the compiler sometimes (depends on the one used) optimises your while loop code to the following :
if (condition) {
while (true) {
// Do nothing
}
}
Refer this link for more. Here is a similar SO question.
edited Nov 21 '18 at 6:29
answered Nov 21 '18 at 6:11
PranjalPranjal
1363
1363
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
add a comment |
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
Thank you very much for letting me know the use of volatile
– Void Young
Nov 25 '18 at 2:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405872%2fwhy-cant-whileflag-end%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CXajebQ8rgVKdvKXURtJ0WPw7rlp7DAWHix0C3TOv2Pu N890JDuwj xF,kfI
try marking the flag as "@Volatile var flag = true"
– Abhi
Nov 21 '18 at 6:09