D3js V4.13 set min max height/width
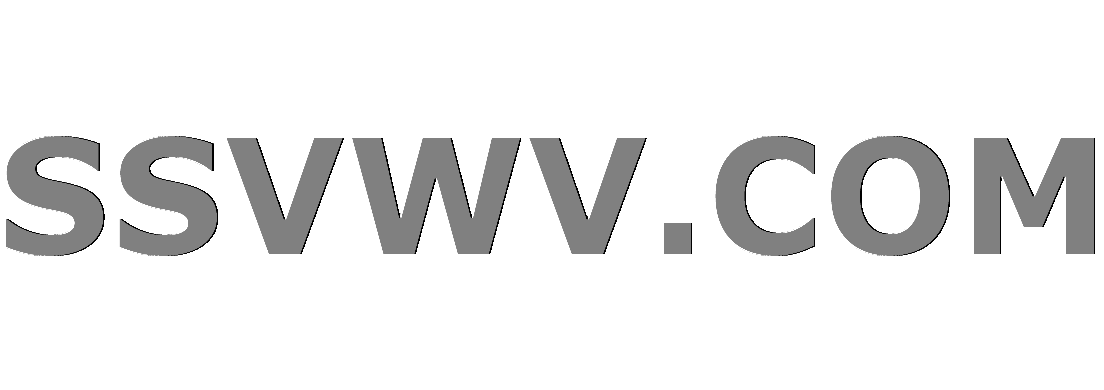
Multi tool use
up vote
0
down vote
favorite
I'm working on a little app to display some data inside circle in d3js
My problem is, sometimes my circles moves outside of the SVG height-width and we can't see the data anymore.
I've been looking for a function which set min-max height-widht, I found one in the version 3 of D3js but not on version 4
I've tried to use forceX and forceY but it center all of my data, I want them to be able to go everywhere in the svg without the gravity impression that forceX and forceY gives
here is a part of my code :
const simulation = forceSimulation()
.force('link', forceLink().id((d) => d['id']).distance(1).strength(1))
.force('charge', forceManyBody())
.force('center', forceCenter(width / 2, height / 2))
.force('collide', forceCollide(d => calculCollide(d)))
json('assets/example.json', (err, data) => {
const link = svg.append('g')
.attr('class', 'links')
.selectAll('line')
.data(data['links'])
.enter()
.append('line')
.attr('stroke-width', (d) => Math.sqrt(d['value']));
const node = svg.append('g')
.attr('class', 'nodes')
.selectAll('circle')
.data(data['nodes'])
.enter()
.append('g')
.attr('class','gcircle')
.append('circle')
.attr('r', (d) => radiusGroup(d))
.attr('fill', (d) => color(d['group']))
.call(drag()
.on('start', dragStarted)
.on('drag', dragged)
.on('end', dragEnded)
);
node.append('title')
.text((d) => d['id']);
let text = svg.selectAll('.gcircle')
.append('text')
.attr("font-family", "sans-serif")
.attr("font-size", "10px")
.text(d => d['id']);
simulation
.nodes(data['nodes'])
.on('tick', ticked);
simulation.force<ForceLink<any, any>>('link')
.links(data['links']);
function ticked() {
link
.attr('x1', function(d) { return d['source'].x; })
.attr('y1', function(d) { return d['source'].y; })
.attr('x2', function(d) { return d['target'].x; })
.attr('y2', function(d) { return d['target'].y; });
node
.attr('cx', function(d) { return d['x']; })
.attr('cy', function(d) { return d['y']; });
text
.attr('dx', d => { return d['x'];})
.attr('dy', d => { return d['y'];});
}
});
function dragStarted(d) {
if (!event.active) { simulation.alphaTarget(0.9).restart(); }
d.fx = d.x;
d.fy = d.y;
}
function dragged(d) {
d.fx = event.x;
d.fy = event.y;
}
function radiusGroup(d) {
if (d.group === 1) {
return 15;
} else {
return 10;
}
}
function dragEnded(d) {
if (!event.active) { simulation.alphaTarget(0); }
d.fx = null;
d.fy = null;
}
function calculCollide(d){
if(d.group === 1) {
return 25;
} else {
return 10;
}
}
PS: I'm using d3js with angular, so I use the @types/d3
here is a stackblitz : https://stackblitz.com/edit/angular-tpbq2t , but with more data circle can go outside of the screen ... and that's my problem ...
javascript css angular d3.js
add a comment |
up vote
0
down vote
favorite
I'm working on a little app to display some data inside circle in d3js
My problem is, sometimes my circles moves outside of the SVG height-width and we can't see the data anymore.
I've been looking for a function which set min-max height-widht, I found one in the version 3 of D3js but not on version 4
I've tried to use forceX and forceY but it center all of my data, I want them to be able to go everywhere in the svg without the gravity impression that forceX and forceY gives
here is a part of my code :
const simulation = forceSimulation()
.force('link', forceLink().id((d) => d['id']).distance(1).strength(1))
.force('charge', forceManyBody())
.force('center', forceCenter(width / 2, height / 2))
.force('collide', forceCollide(d => calculCollide(d)))
json('assets/example.json', (err, data) => {
const link = svg.append('g')
.attr('class', 'links')
.selectAll('line')
.data(data['links'])
.enter()
.append('line')
.attr('stroke-width', (d) => Math.sqrt(d['value']));
const node = svg.append('g')
.attr('class', 'nodes')
.selectAll('circle')
.data(data['nodes'])
.enter()
.append('g')
.attr('class','gcircle')
.append('circle')
.attr('r', (d) => radiusGroup(d))
.attr('fill', (d) => color(d['group']))
.call(drag()
.on('start', dragStarted)
.on('drag', dragged)
.on('end', dragEnded)
);
node.append('title')
.text((d) => d['id']);
let text = svg.selectAll('.gcircle')
.append('text')
.attr("font-family", "sans-serif")
.attr("font-size", "10px")
.text(d => d['id']);
simulation
.nodes(data['nodes'])
.on('tick', ticked);
simulation.force<ForceLink<any, any>>('link')
.links(data['links']);
function ticked() {
link
.attr('x1', function(d) { return d['source'].x; })
.attr('y1', function(d) { return d['source'].y; })
.attr('x2', function(d) { return d['target'].x; })
.attr('y2', function(d) { return d['target'].y; });
node
.attr('cx', function(d) { return d['x']; })
.attr('cy', function(d) { return d['y']; });
text
.attr('dx', d => { return d['x'];})
.attr('dy', d => { return d['y'];});
}
});
function dragStarted(d) {
if (!event.active) { simulation.alphaTarget(0.9).restart(); }
d.fx = d.x;
d.fy = d.y;
}
function dragged(d) {
d.fx = event.x;
d.fy = event.y;
}
function radiusGroup(d) {
if (d.group === 1) {
return 15;
} else {
return 10;
}
}
function dragEnded(d) {
if (!event.active) { simulation.alphaTarget(0); }
d.fx = null;
d.fy = null;
}
function calculCollide(d){
if(d.group === 1) {
return 25;
} else {
return 10;
}
}
PS: I'm using d3js with angular, so I use the @types/d3
here is a stackblitz : https://stackblitz.com/edit/angular-tpbq2t , but with more data circle can go outside of the screen ... and that's my problem ...
javascript css angular d3.js
what have you found in D3v3?
– rioV8
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
from the docsThe size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.
– rioV8
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm working on a little app to display some data inside circle in d3js
My problem is, sometimes my circles moves outside of the SVG height-width and we can't see the data anymore.
I've been looking for a function which set min-max height-widht, I found one in the version 3 of D3js but not on version 4
I've tried to use forceX and forceY but it center all of my data, I want them to be able to go everywhere in the svg without the gravity impression that forceX and forceY gives
here is a part of my code :
const simulation = forceSimulation()
.force('link', forceLink().id((d) => d['id']).distance(1).strength(1))
.force('charge', forceManyBody())
.force('center', forceCenter(width / 2, height / 2))
.force('collide', forceCollide(d => calculCollide(d)))
json('assets/example.json', (err, data) => {
const link = svg.append('g')
.attr('class', 'links')
.selectAll('line')
.data(data['links'])
.enter()
.append('line')
.attr('stroke-width', (d) => Math.sqrt(d['value']));
const node = svg.append('g')
.attr('class', 'nodes')
.selectAll('circle')
.data(data['nodes'])
.enter()
.append('g')
.attr('class','gcircle')
.append('circle')
.attr('r', (d) => radiusGroup(d))
.attr('fill', (d) => color(d['group']))
.call(drag()
.on('start', dragStarted)
.on('drag', dragged)
.on('end', dragEnded)
);
node.append('title')
.text((d) => d['id']);
let text = svg.selectAll('.gcircle')
.append('text')
.attr("font-family", "sans-serif")
.attr("font-size", "10px")
.text(d => d['id']);
simulation
.nodes(data['nodes'])
.on('tick', ticked);
simulation.force<ForceLink<any, any>>('link')
.links(data['links']);
function ticked() {
link
.attr('x1', function(d) { return d['source'].x; })
.attr('y1', function(d) { return d['source'].y; })
.attr('x2', function(d) { return d['target'].x; })
.attr('y2', function(d) { return d['target'].y; });
node
.attr('cx', function(d) { return d['x']; })
.attr('cy', function(d) { return d['y']; });
text
.attr('dx', d => { return d['x'];})
.attr('dy', d => { return d['y'];});
}
});
function dragStarted(d) {
if (!event.active) { simulation.alphaTarget(0.9).restart(); }
d.fx = d.x;
d.fy = d.y;
}
function dragged(d) {
d.fx = event.x;
d.fy = event.y;
}
function radiusGroup(d) {
if (d.group === 1) {
return 15;
} else {
return 10;
}
}
function dragEnded(d) {
if (!event.active) { simulation.alphaTarget(0); }
d.fx = null;
d.fy = null;
}
function calculCollide(d){
if(d.group === 1) {
return 25;
} else {
return 10;
}
}
PS: I'm using d3js with angular, so I use the @types/d3
here is a stackblitz : https://stackblitz.com/edit/angular-tpbq2t , but with more data circle can go outside of the screen ... and that's my problem ...
javascript css angular d3.js
I'm working on a little app to display some data inside circle in d3js
My problem is, sometimes my circles moves outside of the SVG height-width and we can't see the data anymore.
I've been looking for a function which set min-max height-widht, I found one in the version 3 of D3js but not on version 4
I've tried to use forceX and forceY but it center all of my data, I want them to be able to go everywhere in the svg without the gravity impression that forceX and forceY gives
here is a part of my code :
const simulation = forceSimulation()
.force('link', forceLink().id((d) => d['id']).distance(1).strength(1))
.force('charge', forceManyBody())
.force('center', forceCenter(width / 2, height / 2))
.force('collide', forceCollide(d => calculCollide(d)))
json('assets/example.json', (err, data) => {
const link = svg.append('g')
.attr('class', 'links')
.selectAll('line')
.data(data['links'])
.enter()
.append('line')
.attr('stroke-width', (d) => Math.sqrt(d['value']));
const node = svg.append('g')
.attr('class', 'nodes')
.selectAll('circle')
.data(data['nodes'])
.enter()
.append('g')
.attr('class','gcircle')
.append('circle')
.attr('r', (d) => radiusGroup(d))
.attr('fill', (d) => color(d['group']))
.call(drag()
.on('start', dragStarted)
.on('drag', dragged)
.on('end', dragEnded)
);
node.append('title')
.text((d) => d['id']);
let text = svg.selectAll('.gcircle')
.append('text')
.attr("font-family", "sans-serif")
.attr("font-size", "10px")
.text(d => d['id']);
simulation
.nodes(data['nodes'])
.on('tick', ticked);
simulation.force<ForceLink<any, any>>('link')
.links(data['links']);
function ticked() {
link
.attr('x1', function(d) { return d['source'].x; })
.attr('y1', function(d) { return d['source'].y; })
.attr('x2', function(d) { return d['target'].x; })
.attr('y2', function(d) { return d['target'].y; });
node
.attr('cx', function(d) { return d['x']; })
.attr('cy', function(d) { return d['y']; });
text
.attr('dx', d => { return d['x'];})
.attr('dy', d => { return d['y'];});
}
});
function dragStarted(d) {
if (!event.active) { simulation.alphaTarget(0.9).restart(); }
d.fx = d.x;
d.fy = d.y;
}
function dragged(d) {
d.fx = event.x;
d.fy = event.y;
}
function radiusGroup(d) {
if (d.group === 1) {
return 15;
} else {
return 10;
}
}
function dragEnded(d) {
if (!event.active) { simulation.alphaTarget(0); }
d.fx = null;
d.fy = null;
}
function calculCollide(d){
if(d.group === 1) {
return 25;
} else {
return 10;
}
}
PS: I'm using d3js with angular, so I use the @types/d3
here is a stackblitz : https://stackblitz.com/edit/angular-tpbq2t , but with more data circle can go outside of the screen ... and that's my problem ...
javascript css angular d3.js
javascript css angular d3.js
asked 2 days ago


Alann
5310
5310
what have you found in D3v3?
– rioV8
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
from the docsThe size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.
– rioV8
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago
add a comment |
what have you found in D3v3?
– rioV8
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
from the docsThe size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.
– rioV8
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago
what have you found in D3v3?
– rioV8
2 days ago
what have you found in D3v3?
– rioV8
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
from the docs
The size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.– rioV8
2 days ago
from the docs
The size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.– rioV8
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53266135%2fd3js-v4-13-set-min-max-height-width%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Ku5wb 4ddD,LZD0T
what have you found in D3v3?
– rioV8
2 days ago
a .size function after d3.layout.force, where you set height width
– Alann
2 days ago
from the docs
The size affects two aspects of the force-directed layout: the gravitational center, and the initial random position.
It does not constrain the position during the simulation.– rioV8
2 days ago
oh I see, so it's like forceX and forceY in the v4 of d3js, but do you know if there a way to solve my problem ?
– Alann
2 days ago