C Compiler Warnings on pthread_create
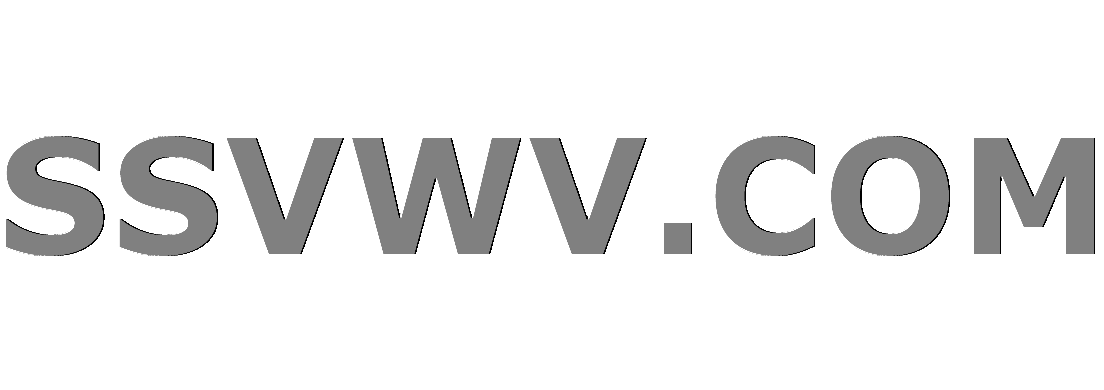
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I don't understand the man page for thread_create. What is a void * nullable? I can't make the array index a global variable for this program. The argument passed to the threads needs to be used in the fibonacci computation. I think somehow I need to get an index (or a pointer to an index) passed and processed by the thread to come up with a fibonacci computation. Here are my warnings:
fibonacci.c:46:31: warning: incompatible pointer types passing 'void *(int)' to parameter of type
'void * _Nullable (* _Nonnull)(void * _Nullable)' [-Wincompatible-pointer-types]
pthread_create(&thread, &a, run, i);
^~~
/usr/include/pthread.h:328:31: note: passing argument to parameter here
void * _Nullable (* _Nonnull)(void * _Nullable),
^
fibonacci.c:46:36: warning: incompatible integer to pointer conversion passing 'int' to parameter
of type 'void *' [-Wint-conversion]
pthread_create(&thread, &a, run, i);
^
/usr/include/pthread.h:329:30: note: passing argument to parameter here
void * _Nullable __restrict);
I originally made the index a global variable but was told that was not allowed because it makes the threads worthless. I'm not sure how else to modify the loop. The point of this program is an exercise in elementary multithreading, so I could use a mutex or semaphore, but I figured joining threads would be the simplest, but I can't figure out how to pass a purposeful argument to the threads. Any ideas? Here is my broken code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(int arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[arg] = 1;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else
{
fibResults[arg] = fibResults[arg -1] + fibResults[arg -2];
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, i);
printf("Thread[%d] createdt", i);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
I'm trying to follow M.M.'s advice and I'm getting new errors and warnings with this code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(void *arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[*arg] = 1;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else
{
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, &fibResults[i]);
printf("Thread[%d] createdt", fibResults[i]);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
Here is what happens when I compile:
gcc -o fibonacci fibonacci.c
fibonacci.c:17:15: error: array subscript is not an integer
fibResults[arg] = 0;
^~~~
fibonacci.c:18:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:22:16: warning: comparison between pointer and integer ('void *' and 'int')
else if (arg == 1)
~~~ ^ ~
fibonacci.c:24:15: error: array subscript is not an integer
fibResults[*arg] = 1;
^~~~~
fibonacci.c:25:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:30:15: error: array subscript is not an integer
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
^~~~~
fibonacci.c:30:40: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:30:62: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:31:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
c multithreading parameter-passing void-pointers
|
show 1 more comment
I don't understand the man page for thread_create. What is a void * nullable? I can't make the array index a global variable for this program. The argument passed to the threads needs to be used in the fibonacci computation. I think somehow I need to get an index (or a pointer to an index) passed and processed by the thread to come up with a fibonacci computation. Here are my warnings:
fibonacci.c:46:31: warning: incompatible pointer types passing 'void *(int)' to parameter of type
'void * _Nullable (* _Nonnull)(void * _Nullable)' [-Wincompatible-pointer-types]
pthread_create(&thread, &a, run, i);
^~~
/usr/include/pthread.h:328:31: note: passing argument to parameter here
void * _Nullable (* _Nonnull)(void * _Nullable),
^
fibonacci.c:46:36: warning: incompatible integer to pointer conversion passing 'int' to parameter
of type 'void *' [-Wint-conversion]
pthread_create(&thread, &a, run, i);
^
/usr/include/pthread.h:329:30: note: passing argument to parameter here
void * _Nullable __restrict);
I originally made the index a global variable but was told that was not allowed because it makes the threads worthless. I'm not sure how else to modify the loop. The point of this program is an exercise in elementary multithreading, so I could use a mutex or semaphore, but I figured joining threads would be the simplest, but I can't figure out how to pass a purposeful argument to the threads. Any ideas? Here is my broken code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(int arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[arg] = 1;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else
{
fibResults[arg] = fibResults[arg -1] + fibResults[arg -2];
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, i);
printf("Thread[%d] createdt", i);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
I'm trying to follow M.M.'s advice and I'm getting new errors and warnings with this code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(void *arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[*arg] = 1;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else
{
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, &fibResults[i]);
printf("Thread[%d] createdt", fibResults[i]);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
Here is what happens when I compile:
gcc -o fibonacci fibonacci.c
fibonacci.c:17:15: error: array subscript is not an integer
fibResults[arg] = 0;
^~~~
fibonacci.c:18:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:22:16: warning: comparison between pointer and integer ('void *' and 'int')
else if (arg == 1)
~~~ ^ ~
fibonacci.c:24:15: error: array subscript is not an integer
fibResults[*arg] = 1;
^~~~~
fibonacci.c:25:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:30:15: error: array subscript is not an integer
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
^~~~~
fibonacci.c:30:40: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:30:62: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:31:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
c multithreading parameter-passing void-pointers
Like the errors say... yourrun()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read thepthread_create()
documentation for the needed types.
– Shawn
Nov 22 '18 at 23:37
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
1
The function must bevoid *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and fromvoid *
. Or you could pass&fibResults[arg]
instead ofarg
.
– M.M
Nov 22 '18 at 23:50
|
show 1 more comment
I don't understand the man page for thread_create. What is a void * nullable? I can't make the array index a global variable for this program. The argument passed to the threads needs to be used in the fibonacci computation. I think somehow I need to get an index (or a pointer to an index) passed and processed by the thread to come up with a fibonacci computation. Here are my warnings:
fibonacci.c:46:31: warning: incompatible pointer types passing 'void *(int)' to parameter of type
'void * _Nullable (* _Nonnull)(void * _Nullable)' [-Wincompatible-pointer-types]
pthread_create(&thread, &a, run, i);
^~~
/usr/include/pthread.h:328:31: note: passing argument to parameter here
void * _Nullable (* _Nonnull)(void * _Nullable),
^
fibonacci.c:46:36: warning: incompatible integer to pointer conversion passing 'int' to parameter
of type 'void *' [-Wint-conversion]
pthread_create(&thread, &a, run, i);
^
/usr/include/pthread.h:329:30: note: passing argument to parameter here
void * _Nullable __restrict);
I originally made the index a global variable but was told that was not allowed because it makes the threads worthless. I'm not sure how else to modify the loop. The point of this program is an exercise in elementary multithreading, so I could use a mutex or semaphore, but I figured joining threads would be the simplest, but I can't figure out how to pass a purposeful argument to the threads. Any ideas? Here is my broken code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(int arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[arg] = 1;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else
{
fibResults[arg] = fibResults[arg -1] + fibResults[arg -2];
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, i);
printf("Thread[%d] createdt", i);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
I'm trying to follow M.M.'s advice and I'm getting new errors and warnings with this code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(void *arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[*arg] = 1;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else
{
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, &fibResults[i]);
printf("Thread[%d] createdt", fibResults[i]);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
Here is what happens when I compile:
gcc -o fibonacci fibonacci.c
fibonacci.c:17:15: error: array subscript is not an integer
fibResults[arg] = 0;
^~~~
fibonacci.c:18:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:22:16: warning: comparison between pointer and integer ('void *' and 'int')
else if (arg == 1)
~~~ ^ ~
fibonacci.c:24:15: error: array subscript is not an integer
fibResults[*arg] = 1;
^~~~~
fibonacci.c:25:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:30:15: error: array subscript is not an integer
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
^~~~~
fibonacci.c:30:40: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:30:62: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:31:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
c multithreading parameter-passing void-pointers
I don't understand the man page for thread_create. What is a void * nullable? I can't make the array index a global variable for this program. The argument passed to the threads needs to be used in the fibonacci computation. I think somehow I need to get an index (or a pointer to an index) passed and processed by the thread to come up with a fibonacci computation. Here are my warnings:
fibonacci.c:46:31: warning: incompatible pointer types passing 'void *(int)' to parameter of type
'void * _Nullable (* _Nonnull)(void * _Nullable)' [-Wincompatible-pointer-types]
pthread_create(&thread, &a, run, i);
^~~
/usr/include/pthread.h:328:31: note: passing argument to parameter here
void * _Nullable (* _Nonnull)(void * _Nullable),
^
fibonacci.c:46:36: warning: incompatible integer to pointer conversion passing 'int' to parameter
of type 'void *' [-Wint-conversion]
pthread_create(&thread, &a, run, i);
^
/usr/include/pthread.h:329:30: note: passing argument to parameter here
void * _Nullable __restrict);
I originally made the index a global variable but was told that was not allowed because it makes the threads worthless. I'm not sure how else to modify the loop. The point of this program is an exercise in elementary multithreading, so I could use a mutex or semaphore, but I figured joining threads would be the simplest, but I can't figure out how to pass a purposeful argument to the threads. Any ideas? Here is my broken code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(int arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[arg] = 1;
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
else
{
fibResults[arg] = fibResults[arg -1] + fibResults[arg -2];
printf("The fibonacci of %d= %dn", arg, fibResults[arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, i);
printf("Thread[%d] createdt", i);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
I'm trying to follow M.M.'s advice and I'm getting new errors and warnings with this code:
#include<stdio.h> //for printf
#include<stdlib.h> //for malloc
#include<pthread.h> //for threading
#define SIZE 25 //number of fibonaccis to be computed
int *fibResults; //array to store fibonacci results
void *run(void *arg) //executes and exits each thread
{
if (arg == 0)
{
fibResults[arg] = 0;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else if (arg == 1)
{
fibResults[*arg] = 1;
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
else
{
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
pthread_exit(0);
}
}
//main function that drives the program.
int main()
{
pthread_attr_t a;
fibResults = (int*)malloc (SIZE * sizeof(int));
pthread_attr_init(&a);
for (int i = 0; i < SIZE; i++)
{
pthread_t thread;
pthread_create(&thread, &a, run, &fibResults[i]);
printf("Thread[%d] createdt", fibResults[i]);
fflush(stdout);
pthread_join(thread, NULL);
printf("Thread[%d] joined & exitedt", i);
}
return 0;
}
Here is what happens when I compile:
gcc -o fibonacci fibonacci.c
fibonacci.c:17:15: error: array subscript is not an integer
fibResults[arg] = 0;
^~~~
fibonacci.c:18:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:22:16: warning: comparison between pointer and integer ('void *' and 'int')
else if (arg == 1)
~~~ ^ ~
fibonacci.c:24:15: error: array subscript is not an integer
fibResults[*arg] = 1;
^~~~~
fibonacci.c:25:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
^~~~~
fibonacci.c:30:15: error: array subscript is not an integer
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
^~~~~
fibonacci.c:30:40: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:30:62: error: invalid operands to binary expression ('void' and 'int')
fibResults[*arg] = fibResults[*arg -1] + fibResults[*arg -2];
~~~~ ^~
fibonacci.c:31:57: error: array subscript is not an integer
printf("The fibonacci of %d= %dn", *arg, fibResults[*arg]);
c multithreading parameter-passing void-pointers
c multithreading parameter-passing void-pointers
edited Nov 23 '18 at 1:11
efuddy
asked Nov 22 '18 at 23:26
efuddyefuddy
495
495
Like the errors say... yourrun()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read thepthread_create()
documentation for the needed types.
– Shawn
Nov 22 '18 at 23:37
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
1
The function must bevoid *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and fromvoid *
. Or you could pass&fibResults[arg]
instead ofarg
.
– M.M
Nov 22 '18 at 23:50
|
show 1 more comment
Like the errors say... yourrun()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read thepthread_create()
documentation for the needed types.
– Shawn
Nov 22 '18 at 23:37
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
1
The function must bevoid *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and fromvoid *
. Or you could pass&fibResults[arg]
instead ofarg
.
– M.M
Nov 22 '18 at 23:50
Like the errors say... your
run()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read the pthread_create()
documentation for the needed types.– Shawn
Nov 22 '18 at 23:37
Like the errors say... your
run()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read the pthread_create()
documentation for the needed types.– Shawn
Nov 22 '18 at 23:37
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
1
1
The function must be
void *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and from void *
. Or you could pass &fibResults[arg]
instead of arg
.– M.M
Nov 22 '18 at 23:50
The function must be
void *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and from void *
. Or you could pass &fibResults[arg]
instead of arg
.– M.M
Nov 22 '18 at 23:50
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439053%2fc-compiler-warnings-on-pthread-create%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439053%2fc-compiler-warnings-on-pthread-create%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n 8u9,w3z JI2rW9ciZ
Like the errors say... your
run()
function has a different type signature than the one needed for a thread entry point, plus you're passing a value of the wrong type to be used as the argument to it. Read thepthread_create()
documentation for the needed types.– Shawn
Nov 22 '18 at 23:37
Compiling on MacOX?
– marko
Nov 22 '18 at 23:40
Yes. I have Mac OSX.
– efuddy
Nov 22 '18 at 23:47
I'm reading the Unix documentation on thread_create and I don't see anything useful. pubs.opengroup.org/onlinepubs/7908799/xsh/pthread_create.html
– efuddy
Nov 22 '18 at 23:49
1
The function must be
void *run(void *arg);
. If you want to pass an integer then you could actually pass the address of the integer and have the run function dereference it. Alternatively (and this is system dependent) you could cast the integer value to and fromvoid *
. Or you could pass&fibResults[arg]
instead ofarg
.– M.M
Nov 22 '18 at 23:50